Embed Quest SDK (Beta)
This article will introduce how to use the Embed SDK to embed frontend components into your website or Mini App.
Live Demo
React SDK: https://codesandbox.io/p/sandbox/mkfdwn
$ npm install @footprint-network/embed-sdk
For Vue or other frameworks, use iframe: https://codesandbox.io/p/devbox/cd4ky2
Usage
Here is a simple React example demonstrating how to use the Embed SDK to embed quest components into your website or Telegram Mini App.
Embed to Website
Once you have created a Quest using the Growthly Admin
, you will receive a Quest ID, which you need to pass to the Quest component.
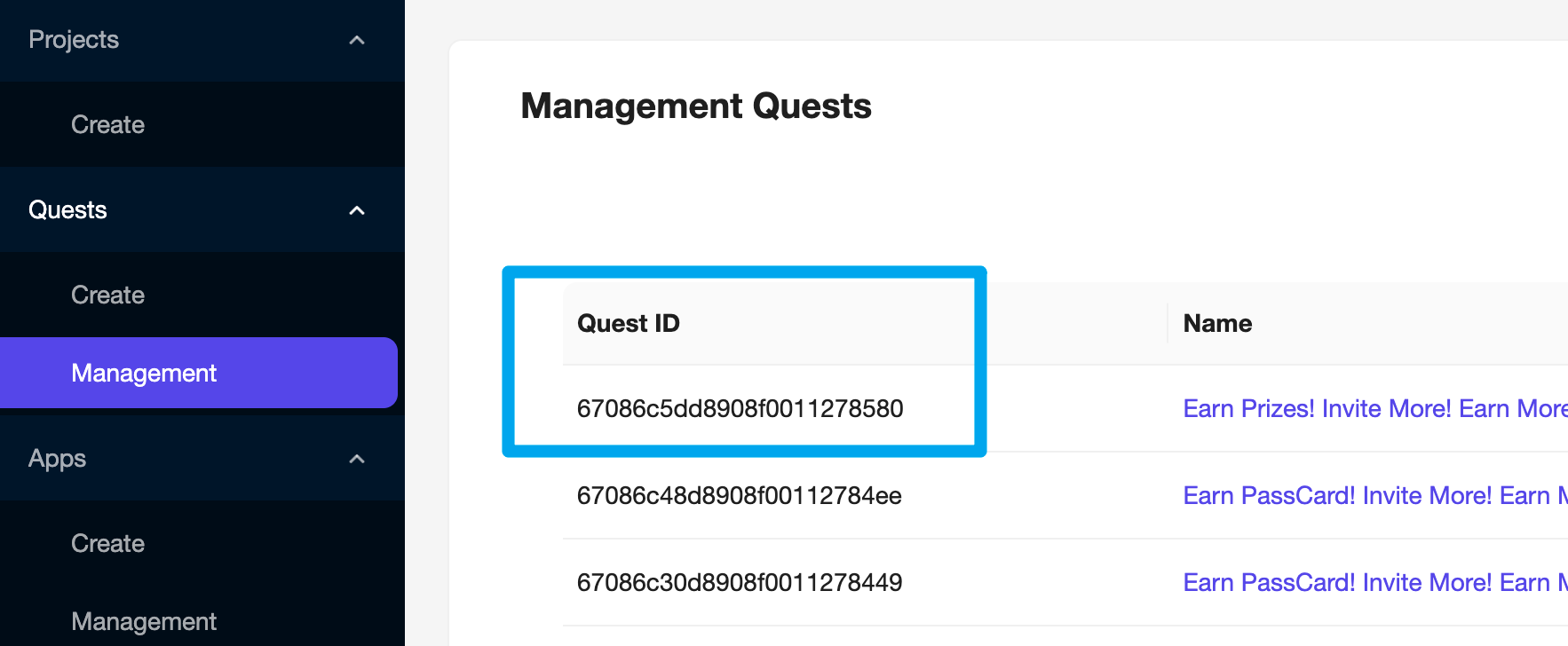
import { Quest } from '@footprint-network/embed-sdk'
export default function App() {
const questId = '67086c5dd8908f0011278580'
return (
<div>
<Quest questId={questId} />
</div>
)
}
Embed to Telegram Mini App
Compared to the Website, Telegram's WebAppInitData parameter provides critical information such as Mini App user details. In your application, you can use methods like location.hash
or this parameter, which you need to pass to the Quest component.
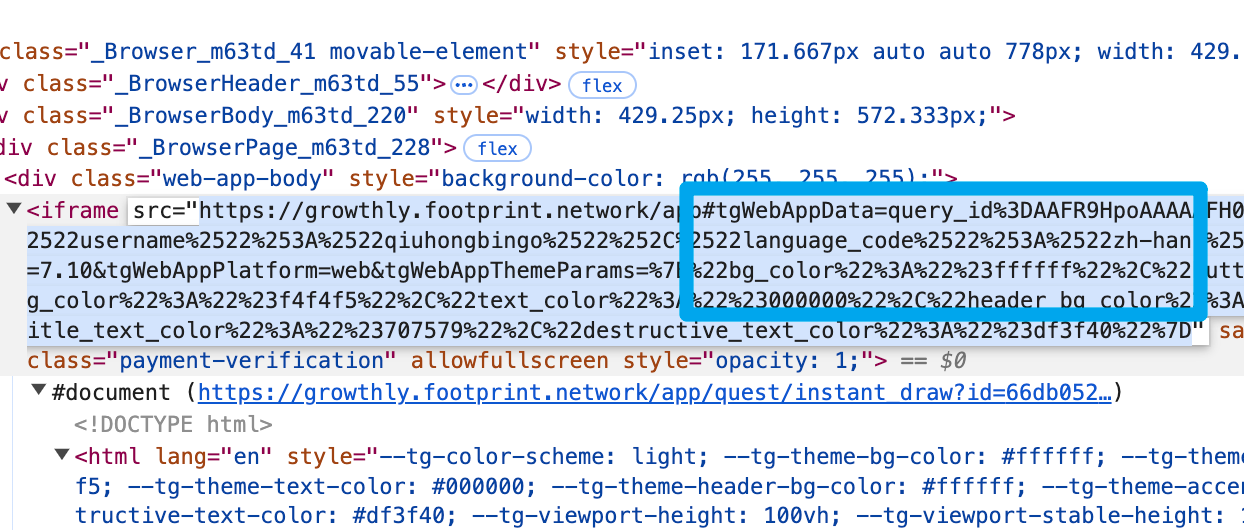
import { Quest } from '@footprint-network/embed-sdk'
export default function App() {
const questId = '67086c5dd8908f0011278580'
const parsedHash = new URLSearchParams(location.hash.substring(1))
const tgWebAppData = encodeURIComponent(parsedHash.get('tgWebAppData'))
// const tgWebAppData = `query_id%3DAAFR9HpoAAAAAFH0emjO4zLX%26user%3D%257B%2522id%2522%253A1752888401%252C%2522first_name%2522%253A%2522Bingo%2520%257C%2520Footprint%2522%252C%2522last_name%2522%253A%2522%2522%252C%2522username%2522%253A%2522qiuhongbingo%2522%252C%2522language_code%2522%253A%2522en%2522%252C%2522allows_write_to_pm%2522%253Atrue%257D%26auth_date%3D1728604189%26hash%3D35a6fc7bcc640ba920e6c6dbcf36476e90c499fcb7d277184319fc0dc3f9960c`
return (
<div>
<Quest questId={questId} tgWebAppData={tgWebAppData} />
</div>
)
}
Test Data
Quest Type | Quest ID | |
---|---|---|
Lucky Draw | lucky_draw | 673c43a92dcc6d0012a634fa |
Cash Rally | cash_rally | 6736b8eb00bfa60012c5fbb5 |
Lucky Wheel | instant_draw | 673da7c122e53100129aaa9d |
Quest | normal | 673da7d822e53100129aaba3 |
Updated 3 months ago